Why doesn't Python have a linked list?
September 22, 2020I recently solved a LeetCode problem with linked lists, which Python doesn't have, so I did some research into:
- What Python does have
- Whether a linked list is faster than Python's options
- When you would need a linked list in Python
No linked lists? Then what does Python have?
Python has native lists that are stored as C arrays, so they have inserts and random access.
Python also has a double-ended queue, the deque (pronounced "deck").
How do deques work?
Data for deque objects is stored in a doubly-linked list of fixed
length blocks. This assures that appends or pops never move any
other data elements besides the one being appended or popped. Python 3.8 source
This means that deques have appends and pops from either end.
Deques have also had an insert function since Python 3.5. Inserts are since they're implemented with the rotate function.
Is a custom linked list faster than Python's deque?
We're going to test Python's deque vs. a custom linked list with:
- Inserts at the beginning.
- Inserts at the middle.
We'll use this minimal linked list implemenetation for testing.
Inserts at the beginning:
For inserts at index , the deque is twice as fast in practice:
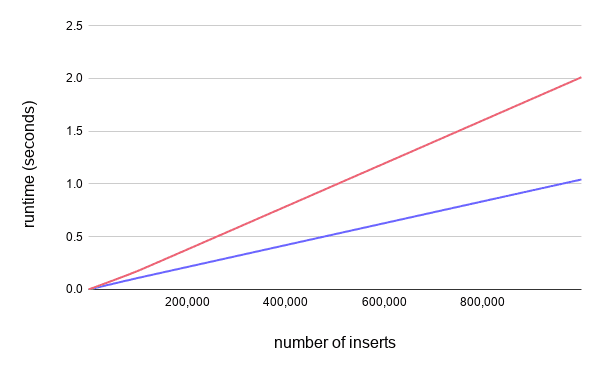
Inserts at the middle:
If we do a fixed number of inserts to the middle, the deque is 100x faster!
size | deque | LinkedList |
---|---|---|
100 | 0.00004 | 0.00126 |
1,000 | 0.00010 | 0.00428 |
10,000 | 0.00043 | 0.04306 |
100,000 | 0.00450 | 0.42688 |
1,000,000 | 0.04525 | 4.34151 |
Custom linked lists can be faster!
Custom linked lists can be faster than deques when you're doing inserts to the middle of the structure. If you already have a reference to a Node, you can insert to the linked list at that location in time.
Deques are built on "fixed length blocks" of multipe elements. An insert to the middle of the structure could overflow a block and force an shift of elements.
When would you need to use a linked list?
A true linked list would only be useful in an algorithm that involves holding onto references to Nodes and manipulating the list with those.
It would require middle-inserts to be a big part of the algorithm in order to overcome the practical speed benefits we saw for the deque.
You might use a linked list to implement other data structures, but the Python language has other solutions for that already:
Python stacks, queues, and heaps are all sets of functions that use native lists as the underlying data structure. |
The synchronized Queue class is built over a deque. |
tl;dr; You rarely need a true linked list in Python and most of the time a deque is faster.